Zend_Validate_Callback Success on False
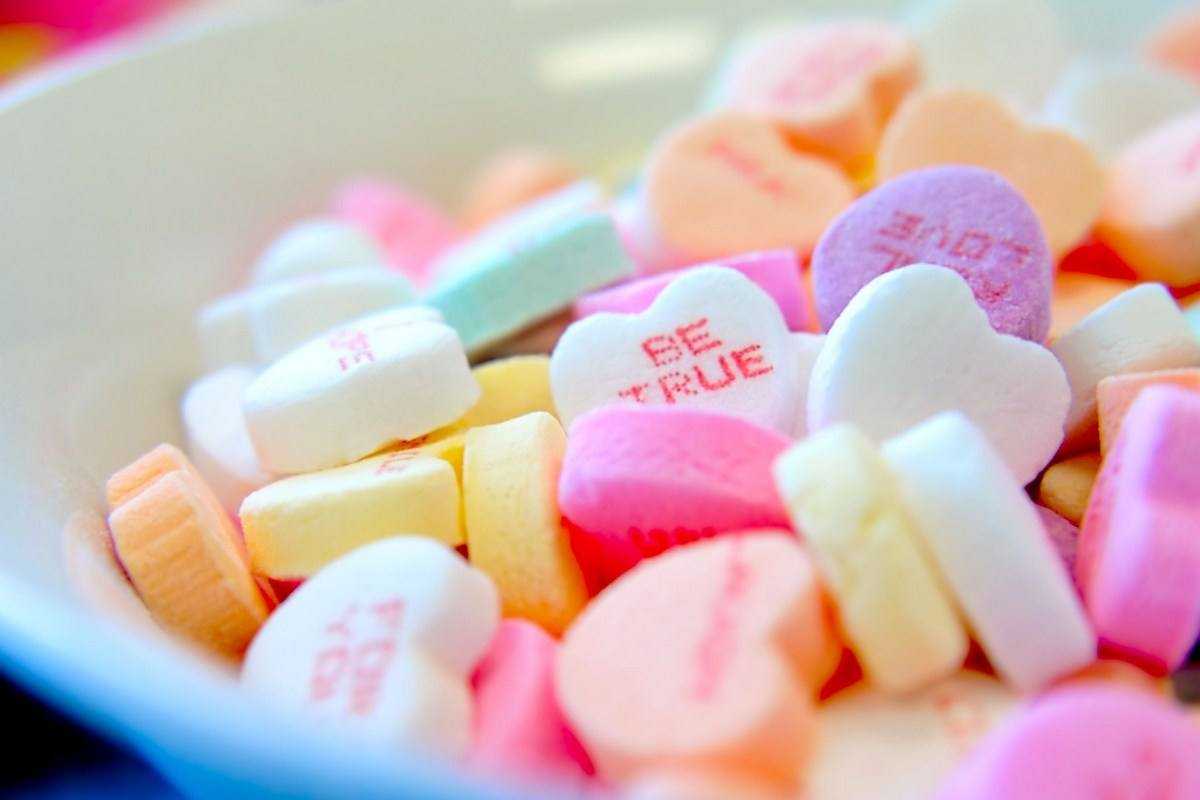
Photo by Obi Onyeador on Unsplash.
What if you need to validate input using a callback function that will return False
when the input is valid? Here's a custom validator to do the job.
class My_Validate_Callback_False extends Zend_Validate_Callback
{
public function isValid($value, $context = NULL)
{
// Run the validator normally and collect the result.
$result = parent::isValid($value, $context);
if($result) {
// The callback returned TRUE, so we should return FALSE.
$this->_error(self::INVALID_VALUE);
return FALSE;
} elseif(in_array(self::INVALID_CALLBACK, $this->_errors)) {
// The callback errored. We should persist the FALSE result.
return FALSE;
} else {
// The callback returned FALSE and did not error. Clear the errors and return TRUE.
$this->_errors = array();
return TRUE;
}
}
}
When would you use this?
If you wrote the callback function, you control the result. But if your callback function is built into PHP or a library, it may not be so easy. You could wrap the function with a version that reverses the result, or you can use My_Validate_Callback_False
.
For example, I have a user registration form with a CAPTCHA. New usernames are checked in the database for uniqueness. But I don't want to query the database unless the user has passed the CAPTCHA — this combats automated queries that probe for usernames. So the username validation chain includes a callback to Zend_Form_Element_Captcha->hasErrors()
.
$username = new Zend_Form_Element_Text('username');
$username->addPrefixPath('My_Validate', 'My/Validate/', 'validate')
->addValidator('Callback_False', TRUE, array(
'callback' => array($captcha, 'hasErrors')));
Because hasErrors()
will return False
when the input is valid, I need My_Validate_Callback_False
to reverse the normal behavior.